总结一下opencv的基础和深入,为了以后的找工作面试可以对答入流,,,太卷了
1 2 3 4 5
| import cv2 import matplotlib.pyplot as plt import numpy as np %matplotlib inline
|
1
| img = cv2.imread('cat.jpeg')
|
array([[[ 27, 69, 34],
[ 24, 66, 31],
[ 21, 63, 28],
...,
[ 96, 159, 109],
[ 98, 161, 111],
[ 94, 160, 109]],
[[ 26, 66, 31],
[ 24, 66, 31],
[ 23, 63, 28],
...,
[ 96, 159, 109],
[ 97, 163, 112],
[ 94, 160, 109]],
[[ 27, 64, 30],
[ 27, 67, 32],
[ 27, 64, 30],
...,
[ 93, 156, 106],
[ 94, 160, 108],
[ 91, 159, 106]],
...,
[[141, 166, 176],
[150, 175, 185],
[139, 164, 174],
...,
[155, 169, 181],
[145, 159, 171],
[129, 143, 155]],
[[140, 166, 173],
[140, 166, 173],
[135, 161, 168],
...,
[189, 203, 215],
[176, 190, 202],
[145, 159, 171]],
[[127, 153, 160],
[137, 163, 170],
[139, 165, 172],
...,
[193, 207, 219],
[169, 183, 195],
[143, 157, 169]]], dtype=uint8)
1 2 3 4 5 6
| def img_show(img): b, g , r = cv2.split(img) img2 = cv2.merge([r, g ,b]) plt.imshow(img2)
|
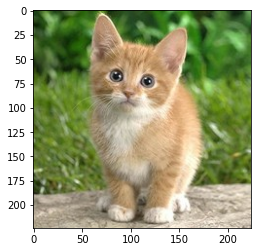
(224, 224, 3)
1 2 3
| img = cv2.imread('cat.jpeg',cv2.IMREAD_GRAYSCALE) img
|
array([[ 54, 51, 48, ..., 137, 139, 137],
[ 51, 51, 48, ..., 137, 140, 137],
[ 50, 52, 50, ..., 134, 137, 135],
...,
[166, 175, 164, ..., 171, 161, 145],
[165, 165, 160, ..., 205, 192, 161],
[152, 162, 164, ..., 209, 185, 159]], dtype=uint8)
(224, 224)
1
| plt.imshow(img, cmap="gray")
|
<matplotlib.image.AxesImage at 0x7fb0dc24f250>
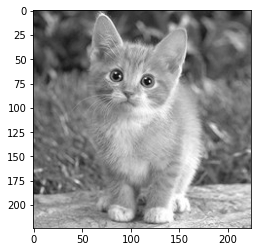
1
| cv2.imwrite('mycat.png',img)
|
True
numpy.ndarray
50176
dtype('uint8')
1
| vc = cv2.VideoCapture('video.mp4')
|
1 2 3 4 5
| if vc.isOpened(): open, frame = vc.read() else: open = False
|
True
1 2 3 4 5 6 7 8 9 10 11 12 13
| while open: ret, frame = vc.read() if frame is None: break if ret ==True: gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
plt.imshow(gray, cmap="gray")
|
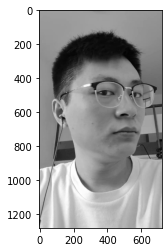
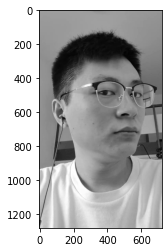
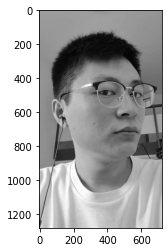
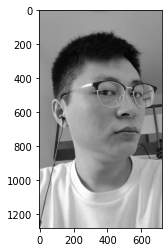
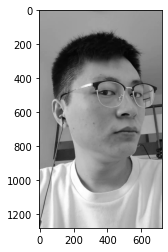
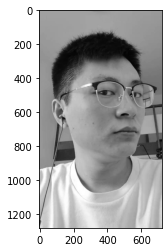
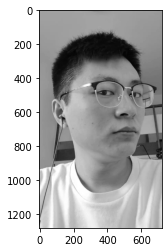
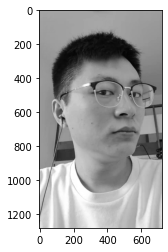
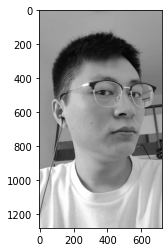
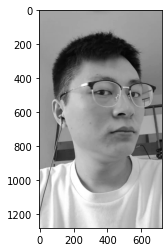
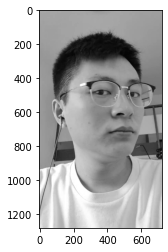
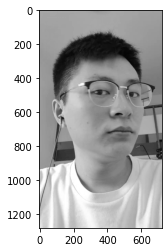
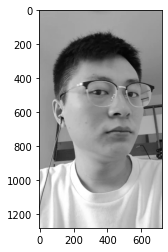
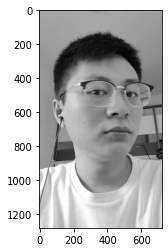
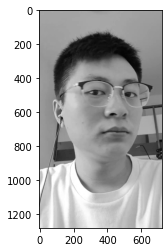
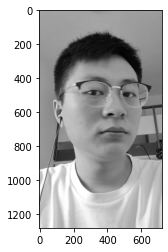
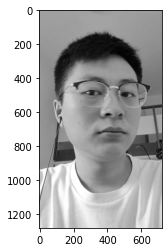
---------------------------------------------------------------------------
KeyboardInterrupt Traceback (most recent call last)
<ipython-input-39-874e08d81013> in <module>
7 # plt.imshow(gray, cmap="gray")# 调用函数显示图片
8 plt.imshow(gray, cmap="gray")
----> 9 plt.pause(1) #显示秒数
10 plt.close()
11 vc.release()
~/anaconda3/envs/cmz/lib/python3.7/site-packages/matplotlib/pyplot.py in pause(interval)
527 canvas.draw_idle()
528 show(block=False)
--> 529 canvas.start_event_loop(interval)
530 else:
531 time.sleep(interval)
~/anaconda3/envs/cmz/lib/python3.7/site-packages/matplotlib/backend_bases.py in start_event_loop(self, timeout)
2457 while self._looping and counter * timestep < timeout:
2458 self.flush_events()
-> 2459 time.sleep(timestep)
2460 counter += 1
2461
KeyboardInterrupt:
截取部分图像数据
1 2 3 4
| img = cv2.imread('cat.jpeg') cat = img[0:200,0:200] img_show(cat)
|
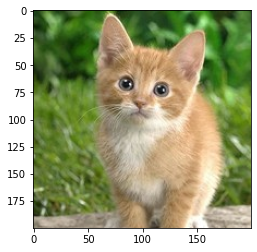
颜色通道的提取
array([[ 69, 66, 63, ..., 159, 161, 160],
[ 66, 66, 63, ..., 159, 163, 160],
[ 64, 67, 64, ..., 156, 160, 159],
...,
[166, 175, 164, ..., 169, 159, 143],
[166, 166, 161, ..., 203, 190, 159],
[153, 163, 165, ..., 207, 183, 157]], dtype=uint8)
(224, 224)
1
| img = cv2.merge((b,g,r))
|
(224, 224, 3)
1 2 3 4 5
| cur_img = img.copy() cur_img[:,:,0] = 0 cur_img[:,:,1] = 0 img_show(cur_img)
|
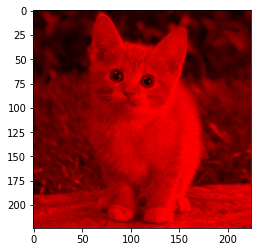
边界填充
1 2 3 4 5 6 7 8
| top_size, bottom_size, left_size,right_size = (50,50,50,50)
replicate = cv2.copyMakeBorder(img, top_size, bottom_size,left_size, right_size, borderType = cv2.BORDER_REPLICATE) reflect = cv2.copyMakeBorder(img, top_size, bottom_size,left_size, right_size, cv2.BORDER_REFLECT) reflect101 = cv2.copyMakeBorder(img, top_size, bottom_size,left_size, right_size, cv2.BORDER_REFLECT_101) wrap = cv2.copyMakeBorder(img, top_size, bottom_size,left_size, right_size, cv2.BORDER_WRAP) constant= cv2.copyMakeBorder(img, top_size, bottom_size,left_size, right_size, cv2.BORDER_CONSTANT, value = 0)
|
1 2 3 4 5 6 7 8 9 10 11 12 13
| plt.subplots_adjust(left=0.225, bottom=0.1, right=2, top=0.9, wspace=0.2, hspace=0.35)
plt.subplot(231), img_show(img),plt.title('ORIGINAL') plt.subplot(232), img_show(replicate),plt.title('replicate') plt.subplot(233), img_show(reflect),plt.title('reflect') plt.subplot(234), img_show(reflect101),plt.title('reflect101') plt.subplot(235), img_show(wrap),plt.title('wrap') plt.subplot(236), img_show(constant),plt.title('constant')
|
(<AxesSubplot:title={'center':'constant'}>, None, Text(0.5, 1.0, 'constant'))
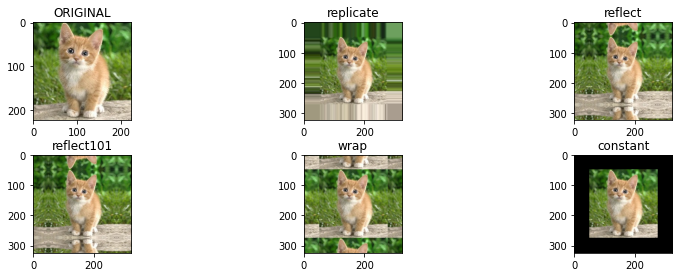
数值计算
1
| img_cat = cv2.imread('cat.jpeg')
|
array([[27, 24, 21, ..., 96, 98, 94],
[26, 24, 23, ..., 96, 97, 94],
[27, 27, 27, ..., 93, 94, 91],
[25, 26, 25, ..., 84, 87, 87],
[28, 24, 22, ..., 78, 82, 82]], dtype=uint8)
array([[ 37, 34, 31, ..., 106, 108, 104],
[ 36, 34, 33, ..., 106, 107, 104],
[ 37, 37, 37, ..., 103, 104, 101],
[ 35, 36, 35, ..., 94, 97, 97],
[ 38, 34, 32, ..., 88, 92, 92]], dtype=uint8)
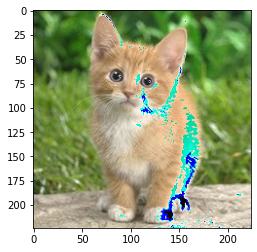
1
| (img_cat2+img_cat)[:5,:,0]
|
array([[ 64, 58, 52, ..., 202, 206, 198],
[ 62, 58, 56, ..., 202, 204, 198],
[ 64, 64, 64, ..., 196, 198, 192],
[ 60, 62, 60, ..., 178, 184, 184],
[ 66, 58, 54, ..., 166, 174, 174]], dtype=uint8)
1
| img_show(img_cat2+img_cat)
|
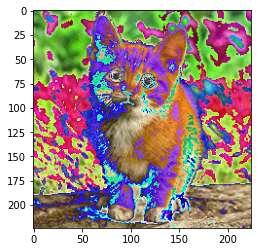
1
| cv2.add(img_cat,img_cat2)[:5,:,0]
|
array([[ 64, 58, 52, ..., 202, 206, 198],
[ 62, 58, 56, ..., 202, 204, 198],
[ 64, 64, 64, ..., 196, 198, 192],
[ 60, 62, 60, ..., 178, 184, 184],
[ 66, 58, 54, ..., 166, 174, 174]], dtype=uint8)
图像融合 图像的shape得是相同的,这里提供了resize
1 2
| img_cat3 = cv2.resize(img_cat,(400,300)) img_cat3.shape
|
(300, 400, 3)
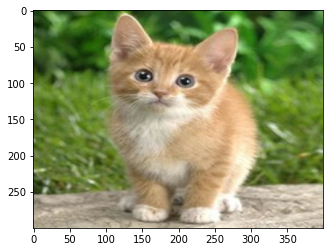
1 2
| img_ca4 = cv2.resize(img_cat,(0,0),fx=3,fy=1) img_show(img_ca4)
|
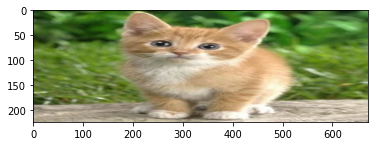
1
| dog = cv2.imread('dog.png')
|
(370, 561, 3)
1
| dog2 = cv2.resize(dog,(224,224))
|
(224, 224, 3)
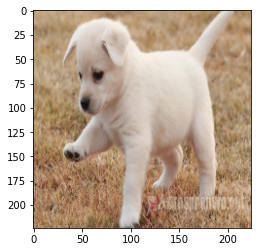
(224, 224, 3)
图像相加是用 R =a倍的x1+ b倍的x2 = ax1 + bx2 + c 偏执项
1
| res = cv2.addWeighted(img_cat,0.4,dog2,0.6,0)
|
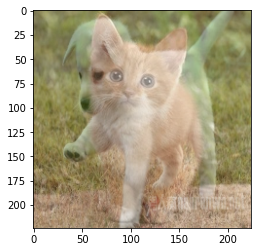